Multipass is pretty useful but what a pain this was to figure out, due to Ubuntu’s Node.js package not working with AWS-CDK.
Multipass lets you manage VM in Ubuntu and can take cloud-init scripts as a parameter. I wanted an Ubuntu LTS instance with AWS CDK, which needs Node.js
and python3-venv
.
#cloud-config
packages:
- python3-venv
- unzip
package_update: true
package_upgrade: true
write_files:
- path: "/etc/environment"
append: true
content: |
export PATH=\
/opt/node-v20.11.1-linux-x64/bin:\
/usr/local/sbin:/usr/local/bin:\
/usr/sbin:/usr/bin:/sbin:/bin:\
/usr/games:/usr/local/games:\
/snap/bin
runcmd:
- wget https://nodejs.org/dist/v20.11.1/node-v20.11.1-linux-x64.tar.xz
- tar xvf node-v20.11.1-linux-x64.tar.xz -C /opt
- export PATH=/opt/node-v20.11.1-linux-x64/bin:$PATH
- npm install -g npm@latest
- npm install -g aws-cdk
- git config --system user.name "Dougie Richardson"
- git config --system user.email "xx@xxxxxxxxx.com"
- git config --system init.defaultBranch main
- wget https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip
- unzip awscli-exe-linux-x86_64.zip
- ./aws/install
Save that as cdk.yaml and spin up an new instance:
multipass launch --name cdk --cloud-init cdk.yaml
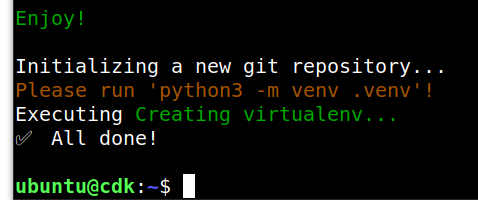
There’s a couple useful things to note if you’re checking this out:
- Inside the VM there’s a useful log to assist debugging
/var/log/cloud-init-output.log
. - While YAML has lots of ways to split text over multiple lines, when you don’t want space use a backslash.
Shell into the new VM with multipass shell cdk
, then we can configure programmatic access and bootstrap CDK.
aws sso configure
aws sso login --profile profile_name
aws sts get-caller-identity --profile profile_name
aws configure get region --profile profile_name
The last two commands give the account and region to bootstrap:
cdk bootstrap aws://account_number/region --profile profile_name